ZealousOtter
New Member
- Feb 17, 2017
- 16
- 11
Hey all. As title suggests, this is a new wired box to close dicemasters. Just like it sounds it will do this:
First, we're going to want to add the CloseDice wired effect. Create a new file CloseDiceBox.cs in the /HabboHotel/Items/Wired/Boxes/Effects/ directory, and add the following:
(CloseDiceBox.cs)
Next, adding the effect we just created to the WiredBoxType.cs file, located in the /HabboHotel/Items/Wired/ directory.
Right below the line:
(WiredBoxType.cs)
Add the new effect:
(WiredBoxType.cs)
We will also need to update the WiredBoxTypeUtility.cs file, found in the /HabboHotel/Items/Wired/ directory:
In the FromWiredId method, below the lines:
(WiredBoxTypeUtility.cs)
Add a new switch for the close dice effect:
(WiredBoxTypeUtility.cs)
Then, in the GetWiredId method, below the line:
(WiredBoxTypeUtility.cs)
Add a new case so that will return '4':
(WiredBoxTypeUtility.cs)
Finally, update the WiredComponent.cs file in the /HabboHotel/Rooms/Instance/ directory:
In the GenerateNewBox method, below the lines:
(WiredComponent.cs)
Add the lines:
(WiredComponent.cs)
Now, just make the effect is being compiled by adding it to Plus Emulator.csproj:
(Plus Emulator.csproj)
Now that the code of the feature is setup, we will just need the furniture for the new wired box. I have made a very simple custom SWF for the necessary functionality (it's not the prettiest thing, but hey, I never claimed to be a designer). Download all of the furni files here:
Add the SWF file to your /dcr/hof_furni/ directory, and add the icon file to the /dcr/hof_furni/icons/ folder. You will also need to add the furnidata provided to your furnidata.xml file, add the productdata to your productdata.txt, and then add the records to the furniture and catalog_items tables in the DB using the SQL inserts. That's all in the download, but here they are as well:
All feedback appreciated. Feel free to edit the code and SWF as needed to your liking.
---------
EDIT: Here is also wired for Freeze and Unfreeze User
First, we're going to want to add the CloseDice wired effect. Create a new file CloseDiceBox.cs in the /HabboHotel/Items/Wired/Boxes/Effects/ directory, and add the following:
(CloseDiceBox.cs)
Code:
using System;
using System.Linq;
using System.Collections.Concurrent;
using Plus.Communication.Packets.Incoming;
using Plus.HabboHotel.Rooms;
namespace Plus.HabboHotel.Items.Wired.Boxes.Effects
{
class CloseDiceBox : IWiredItem, IWiredCycle
{
public Room Instance { get; set; }
public Item Item { get; set; }
public WiredBoxType Type { get { return WiredBoxType.EffectCloseDice; } }
public ConcurrentDictionary<int, Item> SetItems { get; set; }
public int TickCount { get; set; }
public string StringData { get; set; }
public bool BoolData { get; set; }
public int Delay { get { return this._delay; } set { this._delay = value; this.TickCount = value; } }
public string ItemsData { get; set; }
private long _next;
private int _delay = 0;
private bool Requested = false;
public CloseDiceBox(Room Instance, Item Item)
{
this.Instance = Instance;
this.Item = Item;
this.SetItems = new ConcurrentDictionary<int, Item>();
}
public void HandleSave(ClientPacket Packet)
{
this.SetItems.Clear();
int Unknown = Packet.PopInt();
string Unknown2 = Packet.PopString();
int FurniCount = Packet.PopInt();
for (int i = 0; i < FurniCount; i++)
{
Item SelectedItem = Instance.GetRoomItemHandler().GetItem(Packet.PopInt());
if (SelectedItem != null)
SetItems.TryAdd(SelectedItem.Id, SelectedItem);
}
int Delay = Packet.PopInt();
this.Delay = Delay;
}
public bool Execute(params object[] Params)
{
if (this._next == 0 || this._next < PlusEnvironment.Now())
this._next = PlusEnvironment.Now() + this.Delay;
this.Requested = true;
this.TickCount = Delay;
return true;
}
public bool OnCycle()
{
if (this.SetItems.Count == 0 || !Requested)
return false;
long Now = PlusEnvironment.Now();
if (_next < Now)
{
foreach (Item Item in this.SetItems.Values.ToList())
{
if (Item == null)
continue;
if (!Instance.GetRoomItemHandler().GetFloor.Contains(Item))
{
Item n = null;
SetItems.TryRemove(Item.Id, out n);
continue;
}
Item.ExtraData = "0";
Item.UpdateState();
}
Requested = false;
this._next = 0;
this.TickCount = Delay;
}
return true;
}
}
}
Next, adding the effect we just created to the WiredBoxType.cs file, located in the /HabboHotel/Items/Wired/ directory.
Right below the line:
(WiredBoxType.cs)
Code:
EffectExecuteWiredStacks,
Add the new effect:
(WiredBoxType.cs)
Code:
EffectCloseDice,
We will also need to update the WiredBoxTypeUtility.cs file, found in the /HabboHotel/Items/Wired/ directory:
In the FromWiredId method, below the lines:
(WiredBoxTypeUtility.cs)
Code:
case 61:
return WiredBoxType.EffectGiveUserBadge;
Add a new switch for the close dice effect:
(WiredBoxTypeUtility.cs)
Code:
case 62:
return WiredBoxType.EffectCloseDice;
Then, in the GetWiredId method, below the line:
(WiredBoxTypeUtility.cs)
Code:
case WiredBoxType.TriggerStateChanges:
Add a new case so that will return '4':
(WiredBoxTypeUtility.cs)
Code:
case WiredBoxType.EffectCloseDice:
Finally, update the WiredComponent.cs file in the /HabboHotel/Rooms/Instance/ directory:
In the GenerateNewBox method, below the lines:
(WiredComponent.cs)
Code:
case WiredBoxType.EffectMuteTriggerer:
return new MuteTriggererBox(_room, Item);
Add the lines:
(WiredComponent.cs)
Code:
case WiredBoxType.EffectCloseDice:
return new CloseDiceBox(_room, Item);
Now, just make the effect is being compiled by adding it to Plus Emulator.csproj:
(Plus Emulator.csproj)
Code:
<Compile Include="HabboHotel\Items\Wired\Boxes\Effects\CloseDiceBox.cs" />
Now that the code of the feature is setup, we will just need the furniture for the new wired box. I have made a very simple custom SWF for the necessary functionality (it's not the prettiest thing, but hey, I never claimed to be a designer). Download all of the furni files here:
You must be registered for see links
Add the SWF file to your /dcr/hof_furni/ directory, and add the icon file to the /dcr/hof_furni/icons/ folder. You will also need to add the furnidata provided to your furnidata.xml file, add the productdata to your productdata.txt, and then add the records to the furniture and catalog_items tables in the DB using the SQL inserts. That's all in the download, but here they are as well:
(furnidata.xml)
(productdata.txt)
(SQL)
Code:
<furnitype id="49249" classname="wf_act_closes_dices">
<revision>50950</revision>
<defaultdir>0</defaultdir>
<xdim>1</xdim>
<ydim>1</ydim>
<partcolors/>
<name>WIRED Effect: Close Dice</name>
<description>This effect closes the selected Dicemasters.</description>
<adurl></adurl>
<offerid>49249</offerid>
<buyout>1</buyout>
<rentofferid>-1</rentofferid>
<rentbuyout>0</rentbuyout>
<bc>0</bc>
<excludeddynamic>0</excludeddynamic>
<customparams>0</customparams>
<specialtype>1</specialtype>
<canstandon>1</canstandon>
<cansiton>0</cansiton>
<canlayon>0</canlayon>
<furniline>wired</furniline>
</furnitype>
Code:
["wf_act_closes_dices","WIRED Effect: Close Dice","This effect closes the selected Dicemasters."]
Code:
INSERT INTO `furniture` (`id`, `item_name`, `public_name`, `type`, `width`, `length`, `stack_height`, `can_stack`, `can_sit`, `is_walkable`, `sprite_id`, `allow_recycle`, `allow_trade`, `allow_marketplace_sell`, `allow_gift`, `allow_inventory_stack`, `interaction_type`, `interaction_modes_count`, `vending_ids`, `height_adjustable`, `effect_id`, `wired_id`, `is_rare`, `clothing_id`, `extra_rot`)
VALUES
(49249, 'wf_act_closes_dices', 'WIRED Effect: Close Dice', 's', 1, 1, 0.65, '1', '0', '1', 49249, '1', '1', '1', '1', '1', 'wired_effect', 2, '0', '0', 0, 62, '0', 0, '0');
INSERT INTO `catalog_items` (`id`, `page_id`, `item_id`, `catalog_name`, `cost_credits`, `cost_pixels`, `cost_diamonds`, `amount`, `limited_sells`, `limited_stack`, `offer_active`, `extradata`, `badge`, `offer_id`)
VALUES
(49249, 303, '49249', 'WIRED Effect: Close Dice', 3, 0, 0, 1, 0, 0, '1', '', '', 49249);
All feedback appreciated. Feel free to edit the code and SWF as needed to your liking.

---------
EDIT: Here is also wired for Freeze and Unfreeze User
Here are 2 wireds for Freeze User and Unfreeze User.
Screenshots and gif:
Adding the code for these are similar to what we just did for the close dice wired.
Create two new files FreezeUserBox.cs and UnfeezeUserBox.cs in the /HabboHotel/Items/Wired/Boxes/Effects/ directory, and add the following:
(FreezeUserBox.cs)
(UnfreezeUserBox.cs)
Once you have those two files created, do the same changes we did before. In the WiredBoxType.cs file, located in the /HabboHotel/Items/Wired/ directory.
Right below the line (or any line):
(WiredBoxType.cs)
Add the new effects:
(WiredBoxType.cs)
Go to the WiredBoxTypeUtility.cs file, in the /HabboHotel/Items/Wired/ directory.
In the FromWiredId method, below the lines (or would be under EffectGiveUserBadge if close dice isn't added):
(WiredBoxTypeUtility.cs)
Add these lines:
(WiredBoxTypeUtility.cs)
Then, in the same file in the GetWiredId method, below the line:
(WiredBoxTypeUtility.cs)
Add the two new box types:
(WiredBoxTypeUtility.cs)
Update the WiredComponent.cs file in the /HabboHotel/Rooms/Instance/ directory:
In the GenerateNewBox method, below the lines:
(WiredComponent.cs)
Add the lines:
(WiredComponent.cs)
Again, make sure these effects are being compiled by adding them to Plus Emulator.csproj:
(Plus Emulator.csproj)
And again, here are the productdata and SQL inserts for creating the necessary furni. Also, the SWFs that I threw together quickly. Add all this stuff the same way mentioned in the original post.
Screenshots and gif:
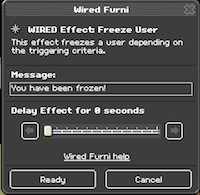
Adding the code for these are similar to what we just did for the close dice wired.
Create two new files FreezeUserBox.cs and UnfeezeUserBox.cs in the /HabboHotel/Items/Wired/Boxes/Effects/ directory, and add the following:
(FreezeUserBox.cs)
Code:
using System;
using System.Linq;
using System.Text;
using System.Collections.Concurrent;
using Plus.Communication.Packets.Incoming;
using Plus.HabboHotel.Rooms;
using Plus.HabboHotel.Users;
using Plus.Communication.Packets.Outgoing.Rooms.Chat;
namespace Plus.HabboHotel.Items.Wired.Boxes.Effects
{
class FreezeUserBox : IWiredItem
{
public Room Instance { get; set; }
public Item Item { get; set; }
public WiredBoxType Type { get { return WiredBoxType.EffectFreezeUser; } }
public ConcurrentDictionary<int, Item> SetItems { get; set; }
public string StringData { get; set; }
public bool BoolData { get; set; }
public string ItemsData { get; set; }
public FreezeUserBox(Room Instance, Item Item)
{
this.Instance = Instance;
this.Item = Item;
this.SetItems = new ConcurrentDictionary<int, Item>();
if (this.SetItems.Count > 0)
this.SetItems.Clear();
}
public void HandleSave(ClientPacket Packet)
{
if (this.SetItems.Count > 0)
this.SetItems.Clear();
int Unknown = Packet.PopInt();
string Message = Packet.PopString();
this.StringData = Message;
Console.Write(Packet.PopInt());
}
public bool Execute(params object[] Params)
{
if (Params.Length != 1)
return false;
Habbo Player = (Habbo)Params[0];
if (Player == null)
return false;
RoomUser User = Instance.GetRoomUserManager().GetRoomUserByHabbo(Player.Id);
Player.GetClient().SendMessage(new WhisperComposer(User.VirtualId, this.StringData, 0, 0));
User.Frozen = true;
return true;
}
}
}
(UnfreezeUserBox.cs)
Code:
using System;
using System.Linq;
using System.Text;
using System.Collections.Concurrent;
using Plus.Communication.Packets.Incoming;
using Plus.HabboHotel.Rooms;
using Plus.HabboHotel.Users;
using Plus.Communication.Packets.Outgoing.Rooms.Chat;
namespace Plus.HabboHotel.Items.Wired.Boxes.Effects
{
class UnfreezeUserBox : IWiredItem
{
public Room Instance { get; set; }
public Item Item { get; set; }
public WiredBoxType Type { get { return WiredBoxType.EffectUnfreezeUser; } }
public ConcurrentDictionary<int, Item> SetItems { get; set; }
public string StringData { get; set; }
public bool BoolData { get; set; }
public string ItemsData { get; set; }
public UnfreezeUserBox(Room Instance, Item Item)
{
this.Instance = Instance;
this.Item = Item;
this.SetItems = new ConcurrentDictionary<int, Item>();
if (this.SetItems.Count > 0)
this.SetItems.Clear();
}
public void HandleSave(ClientPacket Packet)
{
int Unknown = Packet.PopInt();
string Message = Packet.PopString();
this.StringData = Message;
}
public bool Execute(params object[] Params)
{
if (Params.Length != 1)
return false;
Habbo Player = (Habbo)Params[0];
if (Player == null)
return false;
RoomUser User = Instance.GetRoomUserManager().GetRoomUserByHabbo(Player.Id);
Player.GetClient().SendMessage(new WhisperComposer(User.VirtualId, this.StringData, 0, 0));
User.Frozen = false;
return true;
}
}
}
Once you have those two files created, do the same changes we did before. In the WiredBoxType.cs file, located in the /HabboHotel/Items/Wired/ directory.
Right below the line (or any line):
(WiredBoxType.cs)
Code:
EffectCloseDice,
Add the new effects:
(WiredBoxType.cs)
Code:
EffectFreezeUser,
EffectUnfreezeUser,
Go to the WiredBoxTypeUtility.cs file, in the /HabboHotel/Items/Wired/ directory.
In the FromWiredId method, below the lines (or would be under EffectGiveUserBadge if close dice isn't added):
(WiredBoxTypeUtility.cs)
Code:
case 62:
return WiredBoxType.EffectCloseDice;
Add these lines:
(WiredBoxTypeUtility.cs)
Code:
case 63:
return WiredBoxType.EffectFreezeUser;
case 64:
return WiredBoxType.EffectUnfreezeUser;
Then, in the same file in the GetWiredId method, below the line:
(WiredBoxTypeUtility.cs)
Code:
case WiredBoxType.EffectSetRollerSpeed:
Add the two new box types:
(WiredBoxTypeUtility.cs)
Code:
case WiredBoxType.EffectFreezeUser:
case WiredBoxType.EffectUnfreezeUser:
Update the WiredComponent.cs file in the /HabboHotel/Rooms/Instance/ directory:
In the GenerateNewBox method, below the lines:
(WiredComponent.cs)
Code:
case WiredBoxType.EffectMuteTriggerer:
return new MuteTriggererBox(_room, Item);
Add the lines:
(WiredComponent.cs)
Code:
case WiredBoxType.EffectFreezeUser:
return new FreezeUserBox(_room, Item);
case WiredBoxType.EffectUnfreezeUser:
return new UnfreezeUserBox(_room, Item);
Again, make sure these effects are being compiled by adding them to Plus Emulator.csproj:
(Plus Emulator.csproj)
Code:
<Compile Include="HabboHotel\Items\Wired\Boxes\Effects\FreezeUserBox.cs" />
<Compile Include="HabboHotel\Items\Wired\Boxes\Effects\UnfreezeUserBox.cs" />
And again, here are the productdata and SQL inserts for creating the necessary furni. Also, the SWFs that I threw together quickly. Add all this stuff the same way mentioned in the original post.
You must be registered for see links
wf_act_freeze_user
wf_act_unfreeze_us
Code:
--SQL--
INSERT INTO `furniture` (`id`, `item_name`, `public_name`, `type`, `width`, `length`, `stack_height`, `can_stack`, `can_sit`, `is_walkable`, `sprite_id`, `allow_recycle`, `allow_trade`, `allow_marketplace_sell`, `allow_gift`, `allow_inventory_stack`, `interaction_type`, `interaction_modes_count`, `vending_ids`, `height_adjustable`, `effect_id`, `wired_id`, `is_rare`, `clothing_id`, `extra_rot`)
VALUES
(49248, 'wf_act_freeze_user', 'WIRED Effect: Freeze User', 's', 1, 1, 0.65, '1', '0', '1', 49248, '1', '1', '1', '1', '1', 'wired_effect', 2, '0', '0', 0, 63, '0', 0, '0');
INSERT INTO `catalog_items` (`id`, `page_id`, `item_id`, `catalog_name`, `cost_credits`, `cost_pixels`, `cost_diamonds`, `amount`, `limited_sells`, `limited_stack`, `offer_active`, `extradata`, `badge`, `offer_id`)
VALUES
(49248, 303, '49248', 'WIRED Effect: Freeze User', 3, 0, 0, 1, 0, 0, '1', '', '', 49248);
--furnidata.xml--
<furnitype id="49248" classname="wf_act_freeze_user">
<revision>50950</revision>
<defaultdir>0</defaultdir>
<xdim>1</xdim>
<ydim>1</ydim>
<partcolors/>
<name>WIRED Effect: Freeze User</name>
<description>This effect freezes a user depending on the triggering criteria.</description>
<adurl></adurl>
<offerid>-1</offerid>
<buyout>1</buyout>
<rentofferid>-1</rentofferid>
<rentbuyout>0</rentbuyout>
<bc>0</bc>
<excludeddynamic>0</excludeddynamic>
<customparams>0</customparams>
<specialtype>1</specialtype>
<canstandon>1</canstandon>
<cansiton>0</cansiton>
<canlayon>0</canlayon>
<furniline>wired</furniline>
</furnitype>
--productdata.txt--
["wf_act_freeze_user","WIRED Effect: Freeze User","This effect freezes a user depending on the triggering criteria."]
wf_act_unfreeze_us
Code:
--SQL--
INSERT INTO `furniture` (`id`, `item_name`, `public_name`, `type`, `width`, `length`, `stack_height`, `can_stack`, `can_sit`, `is_walkable`, `sprite_id`, `allow_recycle`, `allow_trade`, `allow_marketplace_sell`, `allow_gift`, `allow_inventory_stack`, `interaction_type`, `interaction_modes_count`, `vending_ids`, `height_adjustable`, `effect_id`, `wired_id`, `is_rare`, `clothing_id`, `extra_rot`)
VALUES
(49247, 'wf_act_unfreeze_us', 'WIRED Effect: Unfreeze User', 's', 1, 1, 0.65, '1', '0', '1', 49247, '1', '1', '1', '1', '1', 'wired_effect', 2, '0', '0', 0, 64, '0', 0, '0');
INSERT INTO `catalog_items` (`id`, `page_id`, `item_id`, `catalog_name`, `cost_credits`, `cost_pixels`, `cost_diamonds`, `amount`, `limited_sells`, `limited_stack`, `offer_active`, `extradata`, `badge`, `offer_id`)
VALUES
(49247, 303, '49247', 'WIRED Effect: Unfreeze User', 3, 0, 0, 1, 0, 0, '1', '', '', 49247);
--furnidata.xml--
<furnitype id="49247" classname="wf_act_unfreeze_us">
<revision>50950</revision>
<defaultdir>0</defaultdir>
<xdim>1</xdim>
<ydim>1</ydim>
<partcolors/>
<name>WIRED Effect: Unfreeze User</name>
<description>This effect unfreezes a user depending on the triggering criteria.</description>
<adurl></adurl>
<offerid>-1</offerid>
<buyout>1</buyout>
<rentofferid>-1</rentofferid>
<rentbuyout>0</rentbuyout>
<bc>0</bc>
<excludeddynamic>0</excludeddynamic>
<customparams>0</customparams>
<specialtype>1</specialtype>
<canstandon>1</canstandon>
<cansiton>0</cansiton>
<canlayon>0</canlayon>
<furniline>wired</furniline>
</furnitype>
--productdata.txt--
["wf_act_unfreeze_us","WIRED Effect: Unfreeze User","This effect unfreezes a user depending on the triggering criteria."]
Last edited: