Hello,
First I want to say, this is a hobby project. It will be open source and free, but it isn't meant to be something big. I write this as it's just something I wanted to try out. If it turns out well, I don't mind contributing to the community. If it doesn't, well whatever happens, it is still a good learning experience and loads of fun!
What is Kirsikka?
Kirsikka is the Finnish word for Cherry. It is a Habbo like client written in Javascript (/HTML5, using canvas for rooms mostly). It contains of a server and a client. Both are open source. Tech stuff is written below. It is not the usual client development you see nowadays though (people trying to remake current client), but it's a combination of having oldskool music in a super modern bar: the client will use modern techniques as well as old features, making it fun for R63 lovers as well as oldskool lovers.
Technical stuff client
- Client uses pure JS (so no pixi.js or whatever)
- Client tries to use as little frameworks as possible (things like jQuery will only be used if it's way too much code to do without it, such as animations, draggable plugin etc.)
- Client currently uses sso login, but it might contain an option to enable username(/email) + password login
- Client uses JSON files for configuration and things like texts / variables (or the replacement for it)
Technical stuff server
- Server is written in C# using DotNet Core
- Server uses dependency injection
- Server uses MySQL as database storage
- Server uses Fleck for websockets
Git repository
Keep in mind code is not 100% up to date plus a lot of things have to be done better. More code is here on the thread.
Code snippets
I decided to show code snippets not in the repo, since the rest you can see on your own there.
Images
There's just one cause there's not much to see but expect more, by the way what I got isn't 100% done yet.
Extra info + credits
If you have tips or I do things wrong, PLEASE tell me (and don't say ".... sucks" but tell me how to do it better). I don't mind criticism but if you have it make sure I can learn from it.
Also in case you have any ideas for the client let me know (feature wise for example or something else, let me know)
I want to thank a few people / sites:
- Stackoverflow for a thousand times it helped me out (where would I be without...)
- KittyChloe from ra******** for helping me out with a few things
- Jabbo for a few examples on some stuff
- Google images for a few images like background image
-
First I want to say, this is a hobby project. It will be open source and free, but it isn't meant to be something big. I write this as it's just something I wanted to try out. If it turns out well, I don't mind contributing to the community. If it doesn't, well whatever happens, it is still a good learning experience and loads of fun!
What is Kirsikka?
Kirsikka is the Finnish word for Cherry. It is a Habbo like client written in Javascript (/HTML5, using canvas for rooms mostly). It contains of a server and a client. Both are open source. Tech stuff is written below. It is not the usual client development you see nowadays though (people trying to remake current client), but it's a combination of having oldskool music in a super modern bar: the client will use modern techniques as well as old features, making it fun for R63 lovers as well as oldskool lovers.
Technical stuff client
- Client uses pure JS (so no pixi.js or whatever)
- Client tries to use as little frameworks as possible (things like jQuery will only be used if it's way too much code to do without it, such as animations, draggable plugin etc.)
- Client currently uses sso login, but it might contain an option to enable username(/email) + password login
- Client uses JSON files for configuration and things like texts / variables (or the replacement for it)
Technical stuff server
- Server is written in C# using DotNet Core
- Server uses dependency injection
- Server uses MySQL as database storage
- Server uses Fleck for websockets
Git repository
You must be registered for see links
You must be registered for see links
Keep in mind code is not 100% up to date plus a lot of things have to be done better. More code is here on the thread.
Code snippets
I decided to show code snippets not in the repo, since the rest you can see on your own there.
JavaScript:
import {Credits} from "./items/credits.js";
import {Hotel} from "./items/hotel.js";
export function Toolbar() {
this.items = [];
this.initialize = function() {
this.items.push(new Credits());
this.items.push(new Hotel());
let toolbar = document.createElement('div');
toolbar.id = 'toolbar';
let i = 0;
for (; i < this.items.length; i++) {
const element = document.createElement('div');
element.classList.add('item');
element.title = this.items[i].tooltip;
element.addEventListener('click', this.items[i].onclick);
const imgelement = document.createElement('img');
imgelement.src = this.items[i].icon;
imgelement.alt = this.items[i].alt;
element.appendChild(imgelement);
toolbar.appendChild(element);
}
document.getElementById('game').appendChild(toolbar);
$('.item').tooltip();
};
}
JavaScript:
import {createElement} from "../../utils/dom.js";
export function Credits() {
this.icon = 'images/toolbar/credits.png';
this.tooltip = 'View your currency data';
this.alt = 'credits-icon';
this.onclick = function() {
const element = createElement('div', {'id': 'purse'});
document.getElementById('game').appendChild(element);
/** TODO: Place this in css file */
const textElement = document.createElement('div');
textElement.style.position = 'absolute';
textElement.style.left = '0';
textElement.style.top = '54px';
textElement.style.width = '332px';
textElement.innerHTML = 'You have <span class="creditAmount">' + (window.localStorage.credits||'1000') + '</span> credits';
textElement.style.textAlign = 'center';
textElement.style.fontFamily = 'VolterBold, verdana, arial, sans-serif';
textElement.style.fontSize = '9px';
element.appendChild(textElement);
$(element).draggable();
};
}
Images
There's just one cause there's not much to see but expect more, by the way what I got isn't 100% done yet.
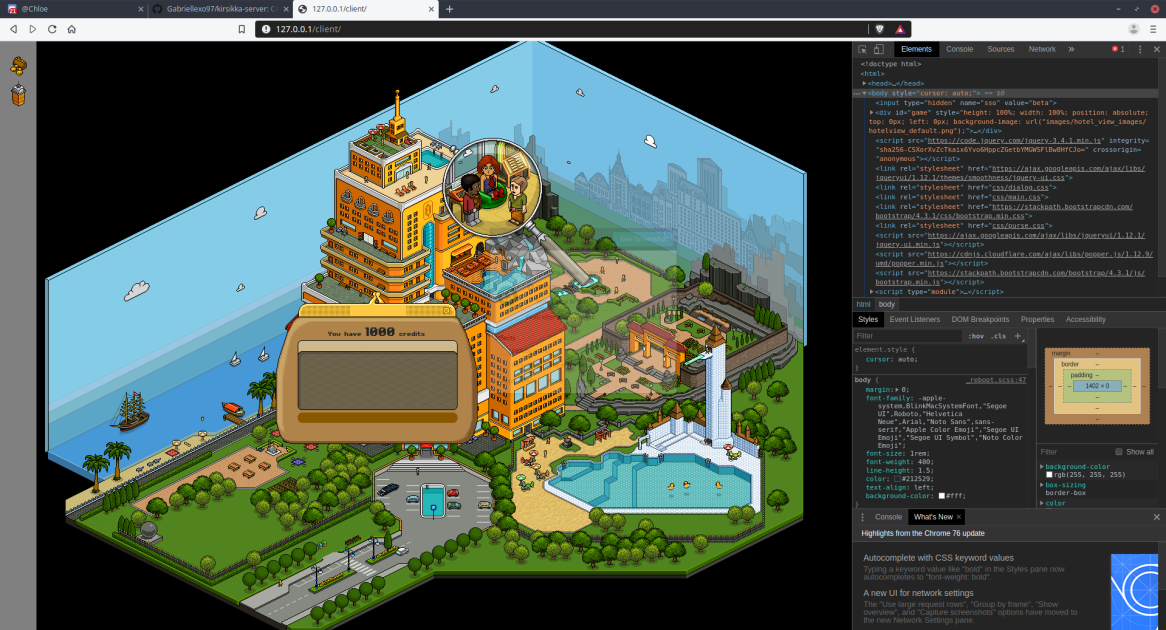
Extra info + credits
If you have tips or I do things wrong, PLEASE tell me (and don't say ".... sucks" but tell me how to do it better). I don't mind criticism but if you have it make sure I can learn from it.
Also in case you have any ideas for the client let me know (feature wise for example or something else, let me know)
I want to thank a few people / sites:
- Stackoverflow for a thousand times it helped me out (where would I be without...)
- KittyChloe from ra******** for helping me out with a few things
- Jabbo for a few examples on some stuff
- Google images for a few images like background image
-
You must be registered for see links
for the Volter & Volter Bold fonts