JynX
Posting Freak
- Feb 6, 2016
- 710
- 438
Hello DevBest, I had a few people ask me if I had a command to add the ability to view a user's PM so I decided to code this and just release it to the public for anyone and everyone to use. This doesn't require hardly ANY knowledge of C# Note: You need to actually have a brain to add this..
It looks like this:
Anyways, here's the code for it (Add this to Plus.HabboHotel.Rooms.Chat.Commands.Moderator):
Then open up your command manager (CommandManager.cs) and find this:
Under that add this:
Then run this query in your database (replace the STAFF ID with the minimum staff rank allowed to have this command):
There you go,
Your welcome,
Jake Adams.
Edit: Added a screenshot.
It looks like this:
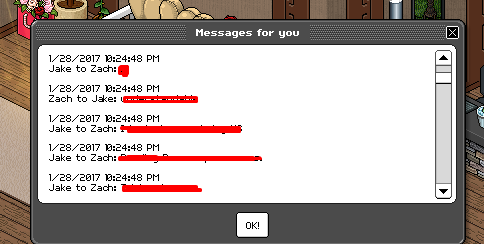
Anyways, here's the code for it (Add this to Plus.HabboHotel.Rooms.Chat.Commands.Moderator):
Code:
using System;
using System.Linq;
using System.Text;
using System.Collections.Generic;
using System.Data;
using Plus.HabboHotel.GameClients;
using Plus.HabboHotel.Rooms;
using Plus.Communication.Packets.Outgoing.Users;
using Plus.Communication.Packets.Outgoing.Rooms.Avatar;
using System.Threading;
using System.Threading.Tasks;
using Plus.Communication.Packets.Outgoing.Rooms.Chat;
using Plus.HabboHotel.Items;
using Plus.HabboHotel.Catalog;
using Plus.Communication.Packets.Outgoing.Inventory.Furni;
using Plus.Database.Interfaces;
using Plus.Communication.Packets.Outgoing.Notifications;
using Plus.Communication.Packets.Outgoing.Rooms.Engine;
namespace Plus.HabboHotel.Rooms.Chat.Commands.Moderator
{
class ViewPrivateMessageCommand : IChatCommand
{
public string PermissionRequired
{
get { return "command_view_pms"; }
}
public string Parameters
{
get { return "%username%"; }
}
public string Description
{
get { return "Lets you view recent PMs from and to a user."; }
}
public void Execute(GameClients.GameClient Session, Rooms.Room Room, string[] Params)
{
if (Params.Length == 1)
{
Session.SendWhisper("Please enter the username of the user you wish to view private messages of.");
return;
}
GameClient TargetClient = PlusEnvironment.GetGame().GetClientManager().GetClientByUsername(Params[1]);
if (TargetClient == null)
{
Session.SendWhisper("An error occoured whilst finding that user, maybe they're not online.");
return;
}
if (TargetClient.GetHabbo() == null)
{
Session.SendWhisper("An error occoured whilst finding that user, maybe they're not online.");
return;
}
StringBuilder b = new StringBuilder();
b.Append("Private messages - " + TargetClient.GetHabbo().Username + "\n\n");
using (IQueryAdapter db = PlusEnvironment.GetDatabaseManager().GetQueryReactor())
{
db.SetQuery("SELECT * FROM `chatlogs_console` WHERE `from_id` = @id OR `to_id` = @id ORDER BY id DESC LIMIT 100");
db.AddParameter("id", TargetClient.GetHabbo().Id);
db.RunQuery();
DataTable t = db.getTable();
foreach (DataRow r in t.Rows)
{
b.Append(UnixTimeStampToDateTime((double)PlusEnvironment.GetUnixTimestamp()) + "\n");
b.Append(getUsernameFromId(Convert.ToInt32(r["from_id"])) + " to " + getUsernameFromId(Convert.ToInt32(r["to_id"])) + ": " + Convert.ToString(r["message"]) + "\n\n");
}
}
Session.SendMessage(new MOTDNotificationComposer(b.ToString()));
}
private DateTime UnixTimeStampToDateTime(double unixTimeStamp)
{
// Unix timestamp is seconds past epoch
System.DateTime dtDateTime = new DateTime(1970, 1, 1, 0, 0, 0, 0, System.DateTimeKind.Utc);
dtDateTime = dtDateTime.AddSeconds(unixTimeStamp).ToLocalTime();
return dtDateTime;
}
private string getUsernameFromId(int userId)
{
if (PlusEnvironment.GetHabboById(userId) != null)
return PlusEnvironment.GetHabboById(userId).Username;
using (IQueryAdapter db = PlusEnvironment.GetDatabaseManager().GetQueryReactor())
{
db.SetQuery("SELECT `username` FROM `users` WHERE `id` = @id");
db.AddParameter("id", userId);
db.RunQuery();
return db.getString();
}
}
}
}
Then open up your command manager (CommandManager.cs) and find this:
Code:
this.Register("ipban", new IPBanCommand());
Code:
this.Register("viewpm", new ViewPrivateMessageCommand());
Then run this query in your database (replace the STAFF ID with the minimum staff rank allowed to have this command):
Code:
INSERT INTO `permissions_commands` VALUES ('command_view_pms', 'STAFF ID', '0');
There you go,
You must be registered for see links
the solution and you're good to go.Your welcome,
Jake Adams.
Edit: Added a screenshot.
Last edited: