Quackster
a devbest user says what
Kepler
What is this?
Kepler is a Habbo Hotel emulator that is designed to fully emulate the v21 version from 2008-ish era. The server is written in Java (JDK 10) and it's using various libraries, which means it's multiplatform, as in supports a wide range of operating systems. Windows, Linux distros, etc. I'm using MariaDB which is a fork of MySQL, so it has the same compatibility but it's more modern.
Libraries Used
- Netty
You must be registered for see links
- HikariCP
You must be registered for see links
- SLF4J
You must be registered for see links
- Log4j
You must be registered for see links
- Apache Commons-Configuration
You must be registered for see links
Features
- User
- Login by SSO ticket.
- Load fuserights.
- Load credits.
- Navigator
- Lists all public rooms .
- Lists all private rooms.
- Navigator category management with rank checking for private rooms.
- Navigator category management with rank checking for public rooms.
- Show recent private rooms created in the categories even if the room owner wasn't online.
- Create private rooms using the navigator.
- Show own rooms.
- Hide room owner name.
- Edit room settings.
- Delete room.
- Messenger
- Search users on console.
- Send user a friend request.
- Accept friend request.
- Reject friend request.
- Send friend message .
- Delete friend.
- Change messenger motto.
- Mark messages are read.
- Show offline messages.
- Follow friend.
- Automatic update friends list.
- Private room
- Walking.
- Walk to door.
- Chat (and message gets worse quality if you're further away from someone in public rooms).
- Shout.
- Whisper.
- Password protect room.
- Public Room
- All possible public rooms added (some may be missing
- All public rooms are fully furnished to what official Habbo had.
- Sitting on furniture in public rooms.
- Lido and Diving Deck
- Change clothes working (with curtain closing).
- Pool lift door closes and opens depending if a user is inside or not.
- Buying tickets work for self and other players.
- Diving.
- Swimming.
- Queue works (line up on first tile and the user automatically walks when there is a free spot).
- Item
- Show own hand (inventory) with items in it.
- Place room items.
- Move and rotate room items.
- Pickup room item.
- Place wall items.
- Pickup wall items.
- Stack items.
- Catalogue
- Show catalogue pages
- Show catalogue items and deals (aka packages)
- Purchase items and packages
- Ranked features
- Add badge automatically if they are a certain rank.
- Command registration checking.
- Commands
- :about.
- :help.
Screenshots
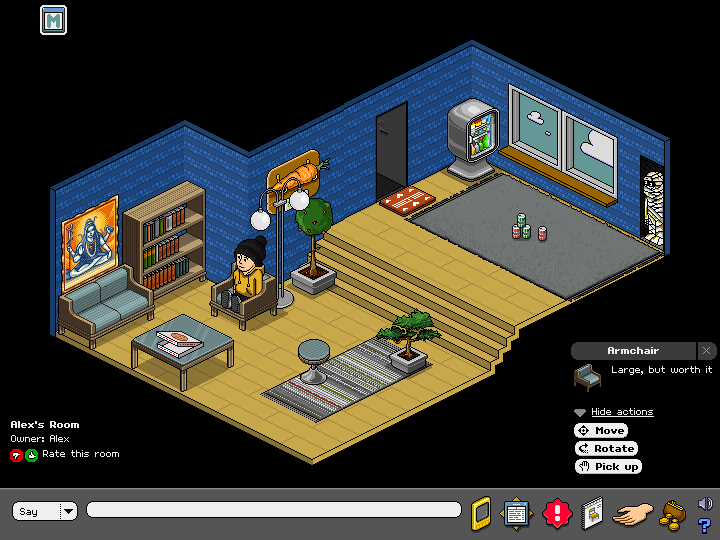
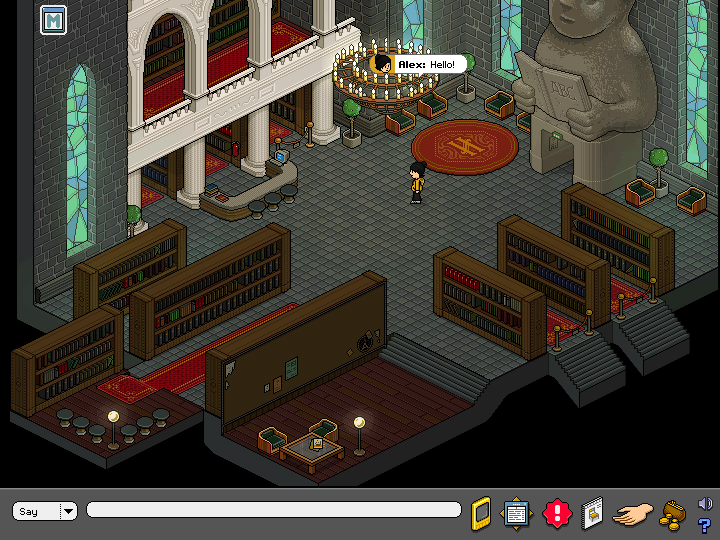
Source code
The source code is available through GithHub at:
You must be registered for see links
.My DCR pack:
You must be registered for see links
Code Snippets
NAVIGATE.java (building the navigator public/private rooms and categories)
Code:
public class NAVIGATE implements MessageEvent {
@Override
public void handle(Player player, NettyRequest reader) {
boolean hideFull = reader.readInt() == 1;
int categoryId = reader.readInt();
NavigatorCategory category = NavigatorManager.getInstance().getCategoryById(categoryId);
if (category == null) {
return;
}
if (category.getMinimumRoleAccess() > player.getDetails().getRank()) {
return;
}
List<NavigatorCategory> subCategories = NavigatorManager.getInstance().getCategoriesByParentId(category.getId());
List<Room> rooms = new ArrayList<>();
int categoryCurrentVisitors = category.getCurrentVisitors();
int categoryMaxVisitors = category.getMaxVisitors();
if (category.isPublicSpaces()) {
for (Room room : RoomManager.getInstance().getRooms()) {
if (room.getData().getCategoryId() != category.getId()) {
continue;
}
if (hideFull && room.getData().getVisitorsNow() >= room.getData().getVisitorsMax()) {
continue;
}
rooms.add(room);
}
} else {
for (Room room : RoomManager.getInstance().replaceQueryRooms(NavigatorDao.getRecentRooms(30, category.getId()))) {
if (room.getData().getCategoryId() != category.getId()) {
continue;
}
if (hideFull && room.getData().getVisitorsNow() >= room.getData().getVisitorsMax()) {
continue;
}
rooms.add(room);
}
}
player.send(new NAVIGATE_LIST(player, category, rooms, hideFull, subCategories, categoryCurrentVisitors, categoryMaxVisitors, player.getDetails().getRank()));
}
}
RoomEntityManager.java snippets
Code:
/**
* Setup handler for the entity to leave room.
*
* [MENTION=2000183830]para[/MENTION]m entity the entity to leave
*/
public void leaveRoom(Entity entity, boolean hotelView) {
if (!this.room.getEntities().contains(entity)) {
return;
}
if (entity.getType() == EntityType.PLAYER) {
PoolHandler.disconnect((Player) entity);
}
RoomTile tile = entity.getRoomUser().getTile();
if (tile != null) {
tile.removeEntity(entity);
}
this.room.getEntities().remove(entity);
this.room.getData().setVisitorsNow(this.room.getEntityManager().getPlayers().size());
this.room.send(new LOGOUT(entity.getRoomUser().getInstanceId()));
this.room.tryDispose(false);
entity.getRoomUser().reset();
// From this point onwards we send packets for the user to leave
if (entity.getType() != EntityType.PLAYER) {
return;
}
Player player = (Player) entity;
if (hotelView) {
player.send(new HOTEL_VIEW());
}
}
Last edited: