Damien
Don't need glasses if you can C#
- Feb 26, 2012
- 434
- 647
This is a command I coded as a response to a help thread that was posted a few days ago which can be found
The command enables you to view other players inventories through your own, while making sure items can't be placed, traded and exchanged in the marketplace. The idea for this command came from @JayCustom, so all credits go to him for this. Enjoy!
Image:
Firstly create a new command and name the file "ViewInventoryCommand.cs" and copy and paste this code into the file:
Inside CommandManager.cs add these commands aswell as any variations of your choosing:
Inside FurniListComposer.cs, replace the code with this:
This is what stops the user, trading; selling or recycling any of the other users items.
Inside PlaceObjectEvent.cs under this line:
Add this segment of code:
In Habbo.cs at the top add this:
Also in Habbo.cs in the Habbo method add this:
Finally in Habbo.cs at the bottom add this:
And there you have it, if I've missed anything please post a comment bellow and I'll add it to the thread.
@GuruGuru, @JayCustom, @Core.
You must be registered for see links
!The command enables you to view other players inventories through your own, while making sure items can't be placed, traded and exchanged in the marketplace. The idea for this command came from @JayCustom, so all credits go to him for this. Enjoy!
Image:
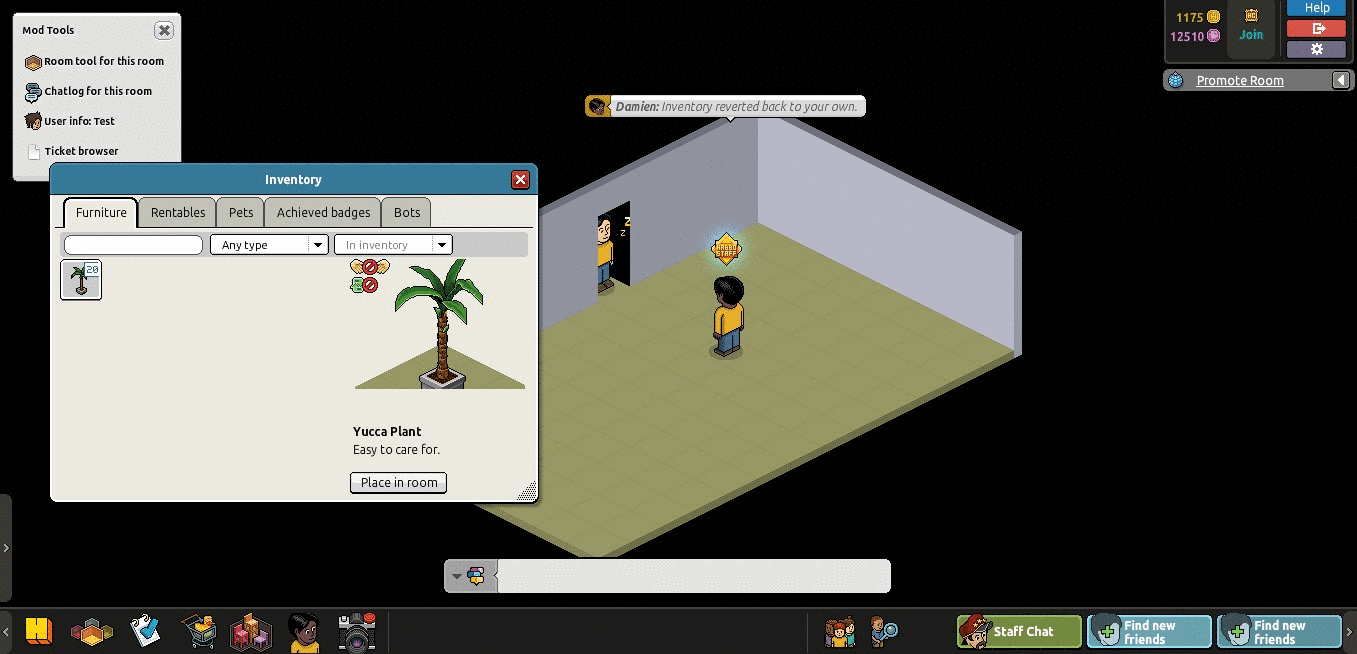
Firstly create a new command and name the file "ViewInventoryCommand.cs" and copy and paste this code into the file:
Code:
using System;
using System.Linq;
using System.Text;
using System.Collections.Generic;
using Plus.HabboHotel.GameClients;
using Plus.Communication.Packets.Outgoing.Inventory.Furni;
namespace Plus.HabboHotel.Rooms.Chat.Commands.Administrator
{
class ViewInventoryCommand : IChatCommand
{
public string PermissionRequired
{
get { return "command_viewinventory"; }
}
public string Parameters
{
get { return ""; }
}
public string Description
{
get { return ""; }
}
public void Execute(GameClient Session, Room Room, string[] Params)
{
if (Session.GetHabbo().ViewInventory)
{
Session.SendMessage(new FurniListComposer(Session.GetHabbo().GetInventoryComponent().GetFloorItems().ToList(), Session.GetHabbo().GetInventoryComponent().GetWallItems()));
Session.GetHabbo().ViewInventory = false;
Session.SendWhisper("Inventory reverted back to your own.");
return;
}
if (Params.Length == 1)
{
Session.SendWhisper("Please enter the username of the user you'd like to check inventory.");
return;
}
GameClient TargetClient = PlusEnvironment.GetGame().GetClientManager().GetClientByUsername(Params[1]);
if (TargetClient == null)
{
Session.SendWhisper("An error occoured whilst finding that user, maybe they're not online.");
return;
}
if (TargetClient.GetHabbo() == null)
{
Session.SendWhisper("An error occoured whilst finding that user, maybe they're not online.");
return;
}
Session.SendMessage(new FurniListComposer(TargetClient.GetHabbo().GetInventoryComponent().GetFloorItems().ToList(), TargetClient.GetHabbo().GetInventoryComponent().GetWallItems(), true));
Session.GetHabbo().ViewInventory = true;
Session.SendWhisper("Next time you open your inventory you'll see " + TargetClient.GetHabbo().Username + "'s inventory.");
}
}
}
Inside CommandManager.cs add these commands aswell as any variations of your choosing:
Code:
this._commands.Add("viewinventory", new ViewInventoryCommand());
this._commands.Add("inv", new ViewInventoryCommand());
Inside FurniListComposer.cs, replace the code with this:
Code:
using System;
using System.Linq;
using System.Text;
using System.Collections.Generic;
using Plus.HabboHotel.Items;
using Plus.HabboHotel.Groups;
using Plus.HabboHotel.Users;
using Plus.HabboHotel.Catalog.Utilities;
namespace Plus.Communication.Packets.Outgoing.Inventory.Furni
{
class FurniListComposer : ServerPacket
{
public FurniListComposer(List<Item> Items, ICollection<Item> Walls, bool StaffCheck = false)
: base(ServerPacketHeader.FurniListMessageComposer)
{
base.WriteInteger(1);
base.WriteInteger(1);
base.WriteInteger(Items.Count + Walls.Count);
foreach (Item Item in Items.ToList())
WriteItem(Item, StaffCheck);
foreach (Item Item in Walls.ToList())
WriteItem(Item, StaffCheck);
}
private void WriteItem(Item Item, bool StaffCheck)
{
base.WriteInteger(Item.Id);
base.WriteString(Item.GetBaseItem().Type.ToString().ToUpper());
base.WriteInteger(Item.Id);
base.WriteInteger(Item.GetBaseItem().SpriteId);
ItemBehaviourUtility.GenerateExtradata(Item, this);
base.WriteBoolean(StaffCheck ? false : Item.GetBaseItem().AllowEcotronRecycle);
base.WriteBoolean(StaffCheck ? false : Item.GetBaseItem().AllowTrade);
base.WriteBoolean(Item.LimitedNo == 0 ? Item.GetBaseItem().AllowInventoryStack : false);
base.WriteBoolean(StaffCheck ? false : ItemUtility.IsRare(Item));
base.WriteInteger(-1);//Seconds to expiration.
base.WriteBoolean(true);
base.WriteInteger(-1);//Item RoomId
if (!Item.IsWallItem)
{
base.WriteString(string.Empty);
base.WriteInteger(0);
}
}
}
}
Inside PlaceObjectEvent.cs under this line:
Code:
if (Session == null || Session.GetHabbo() == null || !Session.GetHabbo().InRoom)
return;
Add this segment of code:
Code:
if (Session.GetHabbo().ViewInventory)
{
Session.SendMessage(new RoomNotificationComposer("furni_placement_error", "message", "This item doesn't belong to you. Are you currently viewing someone elses inventory?"));
return;
}
In Habbo.cs at the top add this:
Code:
private bool _ViewInventory;
Also in Habbo.cs in the Habbo method add this:
Code:
this._ViewInventory = false;
Finally in Habbo.cs at the bottom add this:
Code:
public bool ViewInventory
{
get { return this._ViewInventory; }
set { this._ViewInventory = value; }
}
And there you have it, if I've missed anything please post a comment bellow and I'll add it to the thread.
@GuruGuru, @JayCustom, @Core.