Zeus
Active Member
- Jun 30, 2012
- 150
- 30
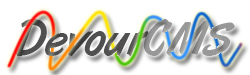
Overview
DevourCMS is a simple, flexible and powerful content management system (CMS). It's built from scratch and provides a very powerful back-end with an extremely easy-to-use front end, as well as keeping security and speed in mind. The CMS will use MySQLi for database related things. It also uses TinyMCE to have the ease of creating pages via the Admin Dashboard.
Features
- Ability to make pages via the Dashboard
- TinyMCE intergration
- MySQLi database engine
- Powerful back-end admin dashboard
- Easy-to-use front end
- Noob friendly
- Installer which does everything for you
- And much, much more...
Snippets and Screenshots
class.version.php:
PHP:
<?php
/*------------------------------------------------------------------------|
| Devour CMS - Copyright (c) Josh Priestley 2012 |
| ------------------------------------------------------------------------|
| Permission is hereby granted, free of charge, to any person obtaining a |
| copy of this software and associated documentation files |
| (the "Software"), to deal in the Software without restriction, |
| including without limitation the rights to use, copy, modify, merge, |
| publish, distribute, sublicense, and/or sell copies of the Software, |
| and to permit persons to whom the Software is furnished to do so, |
| subject to the following conditions: |
| ------------------------------------------------------------------------|
| The above copyright notice and this permission notice shall be included |
| in all copies or substantial portions of the Software. |
| ------------------------------------------------------------------------|
| THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS |
| OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF |
| MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. |
| IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY |
| CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, |
| TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE |
| SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
| ------------------------------------------------------------------------|
/*
* Disable direct access
*/
if(!defined('Devour')) exit ('Direct file access disallowed');
/*
* Begin class for the version system
*/
class dCMS_version
{
/*
* Get the latest version available from an external document
*/
public function getLatest()
{
$version1 = file_get_contents('http://devourcms.com/version.php');
return $version1;
}
/*
* Get the current version the user is using
*/
public function getVersion()
{
$version2 = DEVOUR_VERSION;
return $version2;
}
/*
* Return the latest version
* FALSE - No update
* TRUE - Update avalible
*/
public function checkVersion()
{
$version3 = file_get_contents('http://devourcms.com/version.php');
if($version3 == DEVOUR_VERSION)
{
return false;
}
else
{
return true;
}
}
}
?>
class.core.php (unfinished):
PHP:
<?php
/*------------------------------------------------------------------------|
| Devour CMS - Copyright (c) Josh Priestley 2012 |
| ------------------------------------------------------------------------|
| Permission is hereby granted, free of charge, to any person obtaining a |
| copy of this software and associated documentation files |
| (the "Software"), to deal in the Software without restriction, |
| including without limitation the rights to use, copy, modify, merge, |
| publish, distribute, sublicense, and/or sell copies of the Software, |
| and to permit persons to whom the Software is furnished to do so, |
| subject to the following conditions: |
| ------------------------------------------------------------------------|
| The above copyright notice and this permission notice shall be included |
| in all copies or substantial portions of the Software. |
| ------------------------------------------------------------------------|
| THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS |
| OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF |
| MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. |
| IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY |
| CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, |
| TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE |
| SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
| ------------------------------------------------------------------------|
/*
* Disable direct access
*/
// if(!defined('Devour')) exit ('Direct file access disallowed');
/*
* Require the core files
*/
require_once 'class.user.php';
require_once 'class.manage.php';
require_once 'class.hash.php';
require_once 'inc.config.php';
require_once 'class.version.php';
require_once 'class.template.php';
$core = new dCMS_core();
$user = new dCMS_user();
$hash = new dCMS_hash();
$manage = new dCMS_manage();
$version = new dCMS_version();
$tpl = new dCMS_template();
/*
* Set error reporting
*/
ini_set('display_errors', 'on');
error_reporting(E_ALL);
/*
* Check if the CMS is installed
*/
if($config['install']['value'] == 'Not Installed' && !defined('Devour'))
{
$core->redirect('/_install/step1');
exit;
}
/*
* MySQLi initialisation
*/
$db = new MySQLi
(
$config['mysql']['host'],
$config['mysql']['username'],
$config['mysql']['password'],
$config['mysql']['database']
);
/*
* Maintenance System
*/
if($config['maint']['enabled'] == '1' && !defined('Devour'))
{
$core->Redirect('../maintenance');
exit;
}
/*
* Set the timezone
*/
date_default_timezone_set('Europe/London');
/*
* Set the Devour Version
*/
define('DEVOUR_VERSION','0.0.1');
/*
* Begin class for the core
*/
class dCMS_core
{
public function __construct()
{
global $db;
}
/*
* Redirect function using $core->redirect('URL');
*/
public function redirect($requestUrl)
{
return header('Location: '.$requestUrl);
}
/*
* Using cURL to get the contents of a file
*/
public function get_content($url)
{
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_HEADER, 0);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
$content = curl_exec($ch);
return $content;
curl_close($ch);
}
/*
* Deletes a directory
*/
public function deleteDir($dirPath) {
if (! is_dir($dirPath))
{
$core->redirect('../dir-not-found');
}
if (substr($dirPath, strlen($dirPath) - 1, 1) != '/')
{
$dirPath .= '/';
}
$files = glob($dirPath . '*', GLOB_MARK);
foreach ($files as $file)
{
if (is_dir($file))
{
self::deleteDir($file);
} else {
unlink($file);
}
}
rmdir($dirPath);
}
/*
* Secures a string
*/
public function secure($str)
{
return stripslashes(htmlspecialchars($str));
}
}
?>
class.template.php (unfinished):
PHP:
<?php
/*------------------------------------------------------------------------|
| Devour CMS - Copyright (c) Josh Priestley 2012 |
| ------------------------------------------------------------------------|
| Permission is hereby granted, free of charge, to any person obtaining a |
| copy of this software and associated documentation files |
| (the "Software"), to deal in the Software without restriction, |
| including without limitation the rights to use, copy, modify, merge, |
| publish, distribute, sublicense, and/or sell copies of the Software, |
| and to permit persons to whom the Software is furnished to do so, |
| subject to the following conditions: |
| ------------------------------------------------------------------------|
| The above copyright notice and this permission notice shall be included |
| in all copies or substantial portions of the Software. |
| ------------------------------------------------------------------------|
| THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS |
| OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF |
| MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. |
| IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY |
| CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, |
| TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE |
| SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
| ------------------------------------------------------------------------|
/*
* Begin start of template class
*/
class dCMS_template
{
public $output;
public $file;
public $values = array();
/*
* Functions automatically implemented
*/
public function __construct()
{
global $config, $user;
$this->executeTime = microtime(true);
$this->setParam('updates', $config['update']['enabled']);
$this->setParam('template', $config['site']['template']);
$this->setParam('site_url', $config['site']['url']);
$this->setParam('site_name', $config['site']['name']);
$this->setParam('max_acc', $config['account']['maxAcc']);
$this->setParam('def_rank', $config['account']['defRank']);
$this->setParam('owner', $config['owner']['username']);
$this->setParam('maint_status', $config['maint']['enabled']);
$this->setParam('maint_msgEn', $config['maint']['msgEn']);
$this->setParam('maint_msg', $config['maint']['msg']);
$this->setParam('username', $user->username);
}
/*
* Write a string in the php file
*/
public function write($value)
{
$this->output .= $value;
}
/*
* Begin a .tpl file
*/
public function beginTPL($file)
{
global $core, $config;
$this->file = $file;
if(file_exists('_tpl/'.$this->file.'.tpl'))
{
$str = '';
ob_start();
include('_tpl/'.$this->file.'.tpl');
$str .= ob_get_contents();
ob_end_clean();
$this->output .= $str;
}
else
{
$core->Redirect('../template-error');
exit;
}
}
/*
* Set a param using {param}
*/
public function setParam($key, $value)
{
$this->values[$key] = $value;
}
/*
* Output the page
*/
public function output()
{
foreach($this->values as $key => $value)
{
$tagsToReplace = '{'.$key.'}';
$this->output = str_ireplace($tagsToReplace, $value, $this->output);
}
$this->write('<!--- Devour CMS took '.(microtime(true) - $this->executeTime).'s to load --->');
echo $this->output;
}
}
?>
maintenance.php:
PHP:
<?php
define('Devour','1');
require_once '../../_inc/class.core.php';
if ($db->connect_error)
{
$core->redirect('../mysql_error');
exit;
}
else if ($config['maint']['enabled'] !== '1')
{
$core->redirect('../');
exit;
}
?>
<html>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<link href="../_system/_static/mysql_error.css" rel="stylesheet" type="text/css">
<title><?php echo $config['site']['name']; ?> - Maintenance</title>
<link rel="icon" type="image/vnd.microsoft.icon" href="../_system/_static/_images/favicon.ico">
</head>
<body>
<div id="wrapper">
<div class="box">
<div class="header">
<h1><?php echo $config['site']['name']; ?> is in maintenance!</h1>
<span>
<?php echo $config['site']['name']; ?> is currently in maintenance and is not visible to the public. Please check back later. <?php if ($config['maint']['msgEn'] == '1') { ?>Oh look! A message from the staff here at <?php echo $config['site']['name']; ?>;<br><br><?php echo $config['maint']['msg']; }?>
</span>
</div>
<div class="footer">
<div class="copyright">
© Copyright 2012 · DevourCMS · By Josh Priestley
</div>
</div>
</div>
</div>
</body>
</html>
Devour Original Index:
PHP:
<?php
/*----------------------------------------------|
| ----------- Devour Original Theme ----------- |
|---------------------------------------------- |
| The first theme to come with DevourCMS. |
| developed by Josh Priestley. |
|----------------------------------------------*/
require_once '../../../_inc/class.core.php';
define('Devour','1');
if($user->LoggedIn)
{
$core->redirect('../home');
exit;
}
$tpl->beginTPL('index');
$tpl->setParam('shortDesc', 'We are a site which blogs all the latest news and fasion!');
$tpl->setParam('feed', $plugins->output('Facebook'));
$tpl->output();
?>
Development Status
DevourCMS has no set date to be released. There is a list blow for development status and how far I have gotten with it. The development status list will be changed alot, as the things there are only the things I am working on at the moment.
[x] - Not started
[x] - In the process
[x] - Finished!
class.core.php - [x]
class.users.php - [x]
class.hash.php - [x]
class.version.php - [x]
class.template.php - [x]
inc.config.php - [x]
mysql_error.php - [x]
maintenance.php - [x]
installer - [x]
file structure - [x]
Devour Original Theme - [x]
I will update the above when I begin new files and have new ideas.
Revision Feed (NEW)
Revision 1:
- Began class.template.php
- Added functions to class.core.php
- Started original theme
Revision 2:
- Done more work on class.template.php
- Began original theme pages and styling
- Finished Documentation.php
- Done some .htaccess work
Official Website
DevourCMS now has an official website, which is currently under development and is unfinished, although you can still visit it
You must be registered for see links
Updates
This CMS will come in different versions, 1.0 been the first, there is no last version of this CMS as it will be continued to be developed until I feel the CMS has had enough work. Also, this thread will be updated when I have new snippets and/or screenshots.
Thanks for reading
- Zeus