IntactDev
Member
- Nov 22, 2012
- 399
- 71
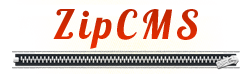
Hello! I'm IntactDev, and you usually wouldn't find me in this section, but I've felt the need to create something that people would use... So, I've decided to make a Habbo CMS! I looked in this development section, and the last development was on March 24th, and that's no bueno, so I'm making a CMS that will compete with the commonly used UberCMS edits.
Feature List:
- TPL System [x]
- News System [x]
- Custom Made Default Theme [x]
- Advanced Backend [x]
Some planned features:
- Ability to buy/sell characters for credits.
- Multiple characters via email.
- News Comments.
- Built-in forums.
- Advanced Banning System (Ban IP, Ban Character, Ban E-Mail, Ban Country, Ban ISP, etc)
Snippets:
common.php
PHP:
<?php
function zipError($title, $desc) {
echo '<style type="text/css">';
echo 'body { margin:0;font-family:Arial, sans-serif;font-size:13px;background:#DDD;color:#1A1A1A; }';
echo 'h1 { color:#FFF;font-family:Arial;font-weight:bold;text-align:center; }';
echo 'h2 { color:#1C1C1C;font-family:Arial;font-weight:bold;text-align:center;padding-bottom:4px;border-bottom:1px solid #AAA; }';
echo '#error#head { background:#1C1C1C;padding:10px;;border-bottom:5px solid #FFF; }';
echo '#error#content { border:1px solid #AAA;background:#FFF;padding:20px;width:780px;margin:30px auto; }';
echo 'p { text-align:center;padding:10px;color:#1A1A1A;font-family:verdana; }';
echo '</style>';
echo '<title>' . $title . '</title>';
echo '<div id="error head"><h1>An error has occurred.</h1></div>';
echo '<div id="error content"><h2>'.$title.'</h2><p>'.$desc.'</p></div>';
}
function secure($string) {
return stripslashes(htmlspecialchars($string));
}
function zipHash($string) {
$hash = md5($string);
$hash = substr($hash, 0, 8);
$hash = md5($hash);
return $hash;
}
?>
config.php
PHP:
<?php
$zip['Site']['Title'] = 'ZipCMS';
$zip['Site']['Location'] = 'http://localhost/ZipCMS'; # Include http:// in the URL #
$zip['Template']['Back'] = 'ZipCMS'; # The default template is ZipCMS #
$zip['Template']['Front'] = 'ZipCMS'; # The default template is ZipCMS #
$zip['Social']['Email'] = '[email protected]'; # E-Mail #
$zip['Social']['Twitter'] = 'IntactDev'; # Twitter #
$zip['Social']['Facebook'] = 'IntactDev'; # Facebook Ending Page URL#
$zip['MySQLi']['Hostname'] = 'localhost';
$zip['MySQLi']['Username'] = 'root';
$zip['MySQLi']['Password'] = 'xxxxxxxxxxxxx';
$zip['MySQLi']['Database'] = 'Zip';
?>
class.zcore.php
PHP:
<?php
class zCore {
final public function getSetting($z) {
global $db;
$q = $db->query('SELECT * FROM `zip_settings` WHERE variable = "' . $z . '"');
while($return = $q->fetch_assoc()) {
$done = $return['value'];
return $done;
}
}
final public function findFunction($z) {
global $users, $zip, $db;
$m = $this->getSetting('maintenance');
if(!isset($z) || empty($z)) {
$z = 'index';
}
if($m == 'false') {
if(!isset($_SESSION['z']['user']['id'])) {
switch($z) {
case 'index':
case null:
case 'lol':
$users->login();
break;
case 'register':
$users->register();
break;
}
} else {
if(!isset($z) || empty($z)) {
$z = 'index';
}
switch($z) {
case 'index':
case 'register':
header("Location: " . $zip['Site']['Location'] . "/home");
break;
}
}
}
}
}
?>
class.ztpl.php
PHP:
<?php
class zTpl {
private $params = Array();
private $tpl;
public function __construct() {
global $zip;
$this->Define('site: title', $zip['Site']['Title']);
$this->Define('site: url', $zip['Site']['Location']);
$this->Define('site: style', $zip['Site']['Location'] . '/_zip/_templates/_front/' . $zip['Template']['Front']);
#$this->Define('stats: online', $this->);
$this->Define('social: email', $zip['Social']['Email']);
$this->Define('social: twitter', $zip['Social']['Twitter']);
$this->Define('social: facebook', $zip['Social']['Facebook']);
}
public function filterParams($param) {
foreach($this->params as $replace => $value) {
$param = str_replace("{" . $replace . "}", $value, $param);
}
return $param;
}
public function Write($str) {
$this->tpl .= $str;
}
public function Define($key, $value) {
$this->params[$key] = $value;
}
public function display() {
echo $this->filterParams($this->tpl);
}
public function addTpl() {
global $zip;
if(!isset($_GET['url']) || empty($_GET['url'])) {
$_GET['url'] = 'index';
}
if(file_exists('_zip/_templates/_front/'. $zip['Template']['Front'] . '/' . secure($_GET['url']) . '.php')) {
ob_start();
include('_zip/_templates/_front/'. $zip['Template']['Front'] . '/' . secure($_GET['url']) . '.php');
$this->tpl .= ob_get_contents();
ob_end_clean();
} else {
die(zipError('File Not Found', 'The file <b>' . secure($_GET['url']) . '</b> could not be found. Please re-check the URL; If you were directed here using a link, please report that link.'));
}
}
}
?>
login code
PHP:
final public function login() {
global $db, $tpl;
$tpl->Define('login: error', null);
if(isset($_POST['login'])) {
if(isset($_POST['username']) && isset($_POST['password'])) {
$username = secure($_POST['username']);
$password = secure($_POST['password']);
$q = $db->query("SELECT * FROM users WHERE username = '{$username}' LIMIT 1");
$num_rows = $q->num_rows;
while($result = $q->fetch_assoc()) {
$dbPassword = $result['password'];
$dbId = $result['id'];
$banned = $result['id'];
}
if($num_rows > 0) {
if(zHash($password) == $dbPassword) {
if($banned == 0) {
$_SESSION['z']['user']['id'] = $dbId;
$_SESSION['z']['user']['username'] = $username;
$_SESSION['z']['logged_in'] = true;
header("Location: /index");
} else {
header("Location: /banned");
}
} else {
$tpl->Define('login: error', '<div id="errmsg" class="cr">The password you entered does not match our records!</div><br />');
}
} else {
$tpl->Define('login: error', '<div id="errmsg" class="cr">The username you have entered has not yet been registered!</div><br />');
}
} else {
$tpl->Define('login: error', '<div id="errmsg" class="cr">Please complete all the fields!</div><br />');
}
}
}
Screenshots:
index
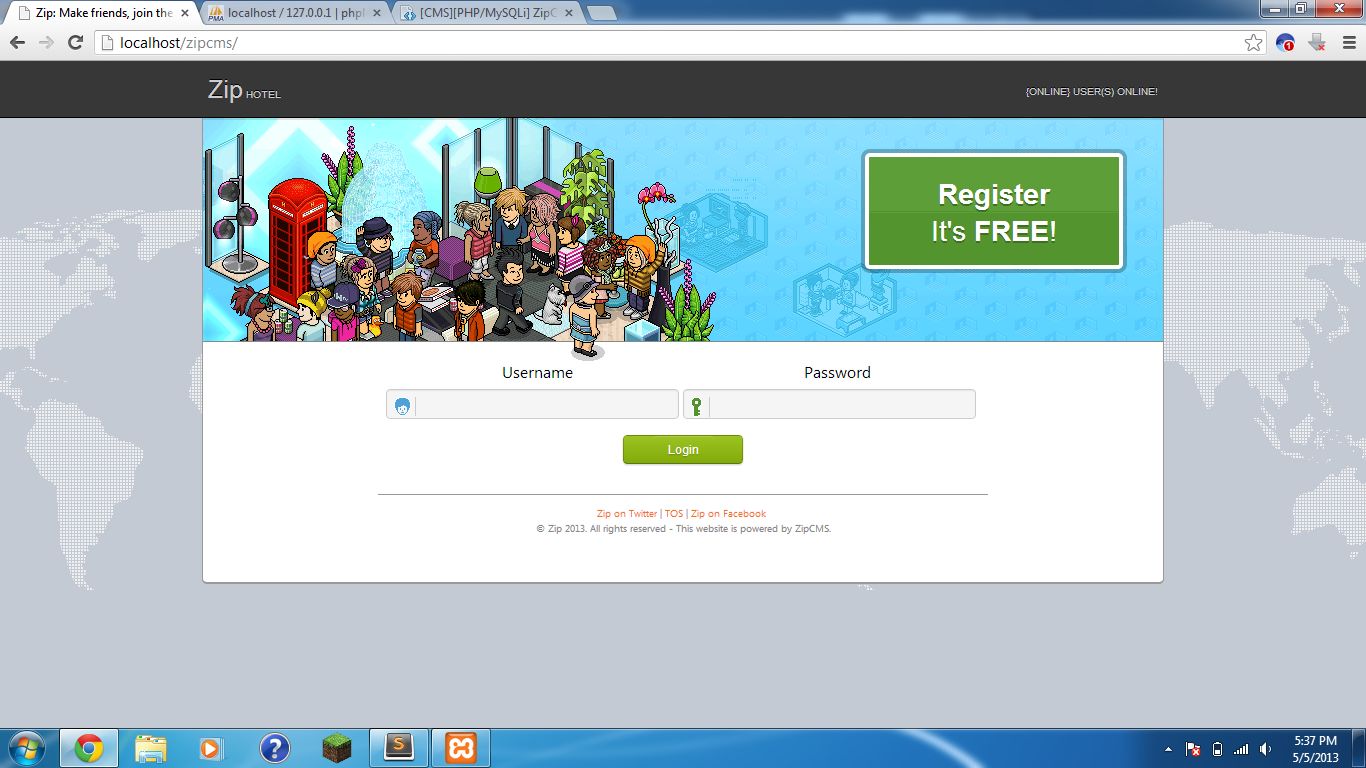
login (error)
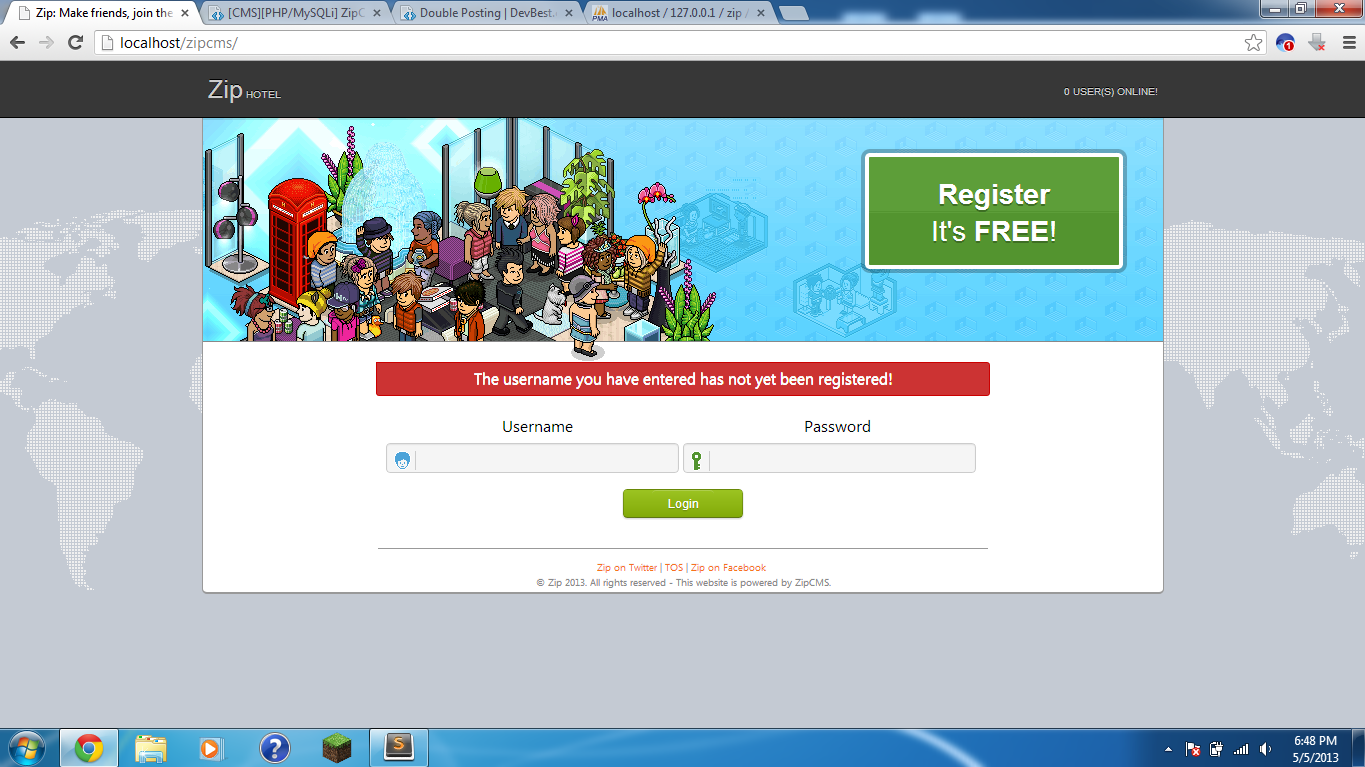
Notes:
The current theme is called "Fuze", which was released as an index, but I will continue it and make all the pages (staff, news, me, register, etc).
Credits:
All my Haters: 99.9% --> For hating, obviously.
Myself: 0.1% --> Coding ZipCMS.